6/2/2025 8:21:00 AM
slxdeveloper.com
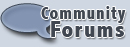 Now Live!
|
|
|
Integrating MindManager With SalesLogix |
|
Description: |
MindManager software allows you to quickly organize data for several uses. Whether to simply display organized data from SalesLogix, or to output action items for an account/contact in SalesLogix to a MindManager document, the possibilities open the door for some quick integration with a great program.
|
Category: |
SalesLogix VBScript Articles
|
Author: |
Ryan Farley
|
Submitted: |
6/16/2005
|
|
|
Stats: |
Article has been read 25706 times
|
Rating:
    - 5.0 out of 5 by 11 users |
|
|
|
fiogf49gjkf0d
MindManager software allows you to quickly organize data for several uses. Whether to simply display organized data from SalesLogix, or to output action items for an account/contact in SalesLogix to a MindManager document, the possibilities open the door for some quick integration with a great program.
MindManager software from MindJet build maps from a central topic with branches to related subjects, issues, tasks, and deliverables; include resources such as live links and documents. It creates organized documents to make brainstorming, design sessions, etc easier and more effective. If you need to do any planning, brain storming, designing, or organizing ideas, MindManager is a great tool to use. I was only recently turned on to MindManager and have made it a permanent part of my routines when brainstorming new ideas, taking notes and action lists from meetings and so forth. I instantly fell in love with this application, from the perspectives of both a user and a developer.
- To learn more, check out the MindJet website
- Visit the MindManager DevZone
MindManager exposes a complete and well thought out object model. Not only does MindManager look like a part of Microsoft Office, but it's object model is so easy to use that you'll forget you're not programming against an Office application. If you're familiar with MindManager, you know that everything comes back to a main central topic. The object model works the same way. You simply create topics that branch off from other topics, all of which lead back to the central topic. Consider the sample below:
Dim mm
Dim doc
Dim t
On Error Resume Next
Set mm = CreateObject("MindManager.Application")
If Err.Number = 429 Then
MsgBox "MindManager is not installed.", 48, "Error"
Exit Sub
End If
On Error Goto 0
mm.Visible = True
Set doc = mm.Documents.Item(0)
With doc.CentralTopic
'create the main topic text
.Text = "Tester Main Topic"
'add sub-topics
.AddSubTopic "Tester 1"
.AddSubTopic "Tester 2"
Set t = .AddSubTopic("Tester 3")
'add sub-topics under the "Tester 3" sub-topic
t.AddSubTopic "Sub Topic 1 of 4"
t.AddSubTopic "Sub Topic 2 of 4"
t.AddSubTopic "Sub Topic 3 of 4"
Set t = t.AddSubTopic("Sub Topic 4 of 4")
'add properties to the "Sub Topic 4 of 4" sub-topic
t.Icons.AddStockIcon 32 'mmStockIconResource1
t.CreateHyperlink "mailto:me@mail.com"
t.Notes.Text = "This is a test to see some notes"
End With
Set t = Nothing
Set doc = Nothing
Set mm = Nothing
This would result in the following MindManager map:
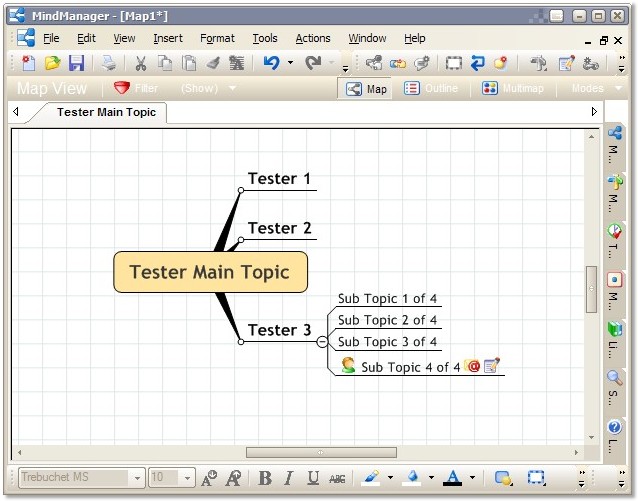
There are several uses where MindManager would make a great enhancement for SalesLogix. A few initial ideas:
- Displaying account hierarchies. A parent account would be represented by the central topic which would branch out to child-accounts.
- Displaying account overview & action items. The account as the central topic branching out to items such as:
- Tickets branching also to associated activities for each ticket.
- Contacts branching out to activities for each contact.
- Opportunities branching out to any activities for the opportunity.
- Importing tasks from a MindManager map to SalesLogix.
- Exporting any custom data, such as projects, campaigns, events, etc to MindManager.
For this article, we will look at simply displaying a map overview of the current account in SalesLogix to keep things simple.
To keep things organized with our code sample, we will construct a VBScript class that can be reused where ever necessary in SalesLogix. This example will export an account overview map from SalesLogix to provide a quick overview of all activities scheduled with contacts from the current account.
Option Explicit
Class AccountMindManager
Private m_accountid
Public Property Let AccountID(ByVal val)
m_accountid = val
End Property
Public Property Get AccountID()
AccountID = m_accountid
End Property
Public Function IsMindManagerInstalled()
Dim mm
On Error Resume Next
Set mm = CreateObject("MindManager.Application")
IsMindManagerInstalled = (Err.Number <> 429)
Set mm = Nothing
On Error Goto 0
End Function
Public Sub CreateDocument()
Dim mm
Dim doc
Dim t
Dim rs
Dim id
If Not IsMindManagerInstalled Then
MsgBox "MindManager is not installed on your computer.", 48, "MindManager Not Installed"
Exit Sub
End If
If Trim(m_accountid) = "" Then
Err.Raise 1000, "AccountID property has not been set."
Exit Sub
End If
Set mm = CreateObject("MindManager.Application")
mm.Visible = True
Set doc = mm.Documents.Add(True)
'add account as central contact
doc.CentralTopic.Text = GetScalarValue("account", "account", "accountid = '" & m_accountid & "'")
'add contacts
Set rs = GetData("contactid, lastname, firstname, email", "contact", "accountid = '" & m_accountid & "'")
With rs
While Not (.BOF Or .EOF)
id = .Fields("contactid").Value
Set t = doc.CentralTopic.AddSubTopic(.Fields("firstname").Value & " " & .Fields("lastname").Value)
t.Icons.AddStockIcon 32 'mmStockIconResource1
If Trim(.Fields("email").Value & "") <> "" Then
t.CreateHyperlink "mailto:" & .Fields("email").Value
End If
AddContactActivities id, t
Set t = Nothing
.MoveNext
Wend
.Close
End With
Set rs = Nothing
Set doc = Nothing
Set mm = Nothing
End Sub
Private Sub AddContactActivities(ByVal ContactID, ByRef ParentTopic)
Dim rs
Dim topic
Dim task
Dim acttype
Dim text
Set rs = GetData("activityid, type, description, startdate, notes", "activity", "contactid = '" & ContactID & "'")
With rs
While Not (.BOF Or .EOF)
acttype = .Fields("type").Value & ""
text = GetTypeText(acttype) & " (" & .Fields("startdate").Value & ") " & .Fields("description").Value
Set topic = ParentTopic.AddSubTopic(text)
topic.Icons.AddStockIcon GetTypeIcon(acttype)
topic.Notes.Text = .Fields("notes").Value & ""
Set task = topic.Task
task.Categories = GetTypeText(acttype)
task.DueDate = .Fields("startdate").Value
task.StartDate = .Fields("startdate").Value
Set task = Nothing
Set topic = Nothing
.MoveNext
Wend
End With
End Sub
Private Function GetScalarValue(ByVal Field, ByVal Table, ByVal Where)
Dim rs
Set rs = Application.GetNewConnection.Execute("select " & Field & " from " & Table & " where " & Where)
With rs
If Not (.BOF Or .EOF) Then
GetScalarValue = .Fields(0).Value & ""
Else
GetScalarValue = ""
End If
.Close
End With
Set rs = Nothing
End Function
Private Function GetData(ByVal Fields, ByVal Table, ByVal Where)
Set GetData = Application.GetNewConnection.Execute("select " & Fields & " from " & Table & " where " & Where)
End Function
Private Function GetTypeText(ByVal ActivityType)
Dim text
Select Case Trim(ActivityType)
Case "262153" text = "Document"
Case "262154" text = "E-Mail"
Case "262155" text = "Fax"
Case "262163" text = "Literature Request"
Case "262145" text = "Meeting"
Case "262148" text = "Note"
Case "262162" text = "Personal Activity"
Case "262146" text = "Phone Call"
Case "262160" text = "Process"
Case "262147" text = "To-Do"
Case Else text = ""
End Select
GetTypeText = text
End Function
Private Function GetTypeIcon(ByVal ActivityType)
Dim ico
Select Case Trim(ActivityType)
Case "262154" ico = 10 'mmStockIconEmail
Case "262155" ico = 42 'mmStockIconFax
Case "262163" ico = 67 'mmStockIconBook
Case "262145" ico = 61 'mmStockIconMeeting
Case "262148" ico = 63 'mmStockIconNote
Case "262146" ico = 40 'mmStockIconCellphone
Case "262147" ico = 62 'mmStockIconCheck
Case Else ico = 67 'mmStockIconBook
End Select
GetTypeIcon = ico
End Function
End Class
To use this class, we could include it in another script, such as one behind a Form and then consume the class as follows:
Dim mm
Set mm = New AccountMindManager
mm.AccountID = Form.CurrentID
mm.CreateDocument
This would result in the following MindManager map:
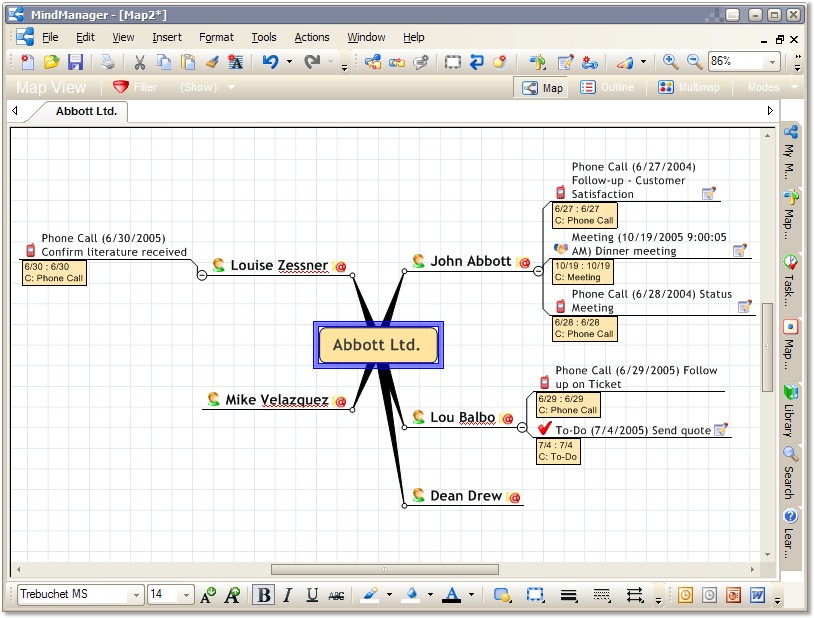
We certainly could go on and on with this idea, adding tickets, opportunities, etc - to the point where we would have a complete overview all in a single map.
Integrating other applications with SalesLogix makes SalesLogix an even more powerful and useful tool. MindManager can enhance the user's experience with SalesLogix like no other application can.
Until next time, happy coding.
-Ryan
|
|
|
|
Rate This Article
|
you must log-in to rate articles. [login here] 
|
|
|
Please log in to rate article. |
|
|
Comments & Discussion
|
you must log-in to add comments. [login here]
|
|
|
- subject is missing.
- comment text is missing.
|
|
| Re: Integrating MindManager With SalesLogix Posted: 6/16/2005 6:41:15 AM | fiogf49gjkf0d GREAT Piece of work Ryan! -- RJLedger | |
|
| Re: Integrating MindManager With SalesLogix Posted: 6/16/2005 7:25:54 AM | fiogf49gjkf0d Oh my god. You did it all in Architect LOL. Great article. I'm checking this product out.
MikeB | |
|
| Re: Integrating MindManager With SalesLogix Posted: 6/27/2005 4:27:02 PM | fiogf49gjkf0d Great Job! I've actually been thinking of doing something like this for a while. You snooze you lose I guess.
How difficult do you think it would be to do the same kind of thing with FreeMind (freemind.sourceforge.net)? The file structure is very simple, I know it wouldn't be too hard. And even though it isn't quite as pretty, you can't beat the price!
Joseph D | |
|
| Re: Integrating MindManager With SalesLogix Posted: 6/27/2005 11:38:37 PM | fiogf49gjkf0d Joseph,
The key is whether or not FreeMind exposes some sort of API or COM layer - or it's file format can be written to in some easy manner. Free is always nice, but while MindJet's MindManager has a high price-tag, it is well worth it IMO. The exposed object model is so complete and very well put together that you feel like you're programming against a uilt in MS Office app. You can get a free viewer app from MindJet also.
That said, it would be interesting to take something free like FreeMind and wrap it in an ActiveX control to display right in SLX forms.
-Ryan | |
|
| Re: Integrating MindManager With SalesLogix Posted: 7/25/2008 1:41:07 AM | fiogf49gjkf0d I've just implemented this and it works a treat - good work Ryan!
Did you ever get round to coding Account Hierarchies? Would be a very nice way of displaying them.
Phil | |
|
| Re: Integrating MindManager With SalesLogix Posted: 7/25/2008 10:58:33 AM | fiogf49gjkf0d Hi Phil,
I'm sure I wrote the code, but can't remember where I did that now (tool long ago). There wouldn't be too many changes from what is shown here in this article.
-Ryan | |
|
|
|
|
|
Visit the slxdeveloper.com Community Forums!
Not finding the information you need here? Try the forums! Get help from others in the community, share your expertise, get what you need from the slxdeveloper.com community. Go to the forums...
|
|
|
|
|
|