6/2/2025 10:21:14 AM
slxdeveloper.com
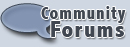 Now Live!
|
|
|
A Guide to The .NET Extension Deployment Attributes |
|
Description: |
When developing .NET Extensions for SalesLogix 7, there are some options available for deployment of your extension. These options are often overlooked, misunderstood, and are certainly undocumented. However, knowing what they are, and how to use them, can assist you to accurately and easily deploy your extensions.
|
Category: |
SalesLogix .NET Extensions Articles
|
Author: |
Ryan Farley
|
Submitted: |
1/23/2007
|
|
|
Stats: |
Article has been read 14303 times
|
Rating:
    - 5.0 out of 5 by 4 users |
|
|
|
fiogf49gjkf0d
When developing .NET Extensions for SalesLogix 7, there are some options available for deployment of your extension. These options are often overlooked, misunderstood, and are certainly undocumented. However, knowing what they are, and how to use them, can assist you to accurately and easily deploy your extensions.
A .NET Extension is deployed the same way that any SalesLogix customization is deployed. It can be bundled and can also synchronize out to remotes. Let's consider the scenario of deploying a non-.NET Extension, a standard SalesLogix Form plugin. In the case of when we bundle a standard Form plugin, we need to also add "supporting items" to the bundle. Things like custom tables, fields, picklists, include scripts, etc. It is up to us to remember to include those items in our project so they are bundled with the Form plugin. The .NET Extensions feature makes this an easier task. There are custom attributes you can use to automatically include other items in the deployment bundle for a .NET Extension. Once you've added these attributes to your .NET Extension, you won't have to worry about forgetting any items in the deployment bundle. The attributes will ensure that the supporting items are included - without you needing to even give it any thought!
An "Attribute" in .NET is like a label you can place on an assembly, class, method, etc to give meaning to the object or code. Think of an Attribute as a label, or modifier, that can describe additional details about your code. These attributes in a .NET Extension are used to describe what supporting plugins, picklist, tables, etc are needed in the bundle for your .NET Extension. Then, when you create the deployment bundle for your extension, the SalesLogix .NET Extension Manager will see the attributes in your .NET Extension's assembly and automatically include the items in the bundle it creates. For .NET Extensions, you can't use just any attribute to describe the supporting items to include in the bundle. The attributes needed for this are defined in the .NET Extensions Framework (Implemented in Sage.SalesLogix.NetExtensions.Framework.dll) and located in the following namespace:
Sage.SalesLogix.NetExtensions.Deployment
There are 15 attributes available that can be used in your .NET Extension assemblies. Each of these attributes can have an optional "Group" number which can be used to indicate the order the items should be included in the deployment bundle if needed. Each of these attributes will translate to an item that can be included in a standard SalesLogix bundle so they should look familiar to you and be somewhat understood by their names alone.
DeployComment
| Description: |
This attribute will place a text comment in the bundle.
| Properties: |
Group(int) - optional, order in bundle Comment(string) - text comment
| Example: |
[assembly: DeployComment("This is my comment")]
|
DeployCopyFiles
| Description: |
Adds a local file to the bundle that will be copied to the specified location on the machine where the bundle is installed.
| Properties: |
Group(int) - optional, order in bundle
SourceDirectory(string) - path on local system (where bundle is created). No file name, directory only
SourceFileSpec(string) - file name in SourceDirectory (this can be a specific file or a wildcard)
TargetDirectory(string) - location (directory only) on target computer (where the bundle is installed) to copy the file(s) to. You can use the same aliases available for any bundle (%App%, %Temp%, %Win%, %Sys%)
| Example: |
[assembly: DeployCopyFiles("C:\\Windows\\Fonts", "*.ttf", "%App%\\Fonts")]
|
DeployCreateField
| Description: |
Creates a new field on an existing table.
| Properties: |
Group(int) - optional, order in bundle
TableName(string) - Name of the table to create the field on
FieldName(string) - Name of the field to create
Note: the tablename.fieldname must exist in the local database
| Example: |
[assembly: DeployCreateField("C_MyTable", "MyField")]
|
DeployCreateIndex
| Description: |
Creates a new index in the target database.
| Properties: |
Group(int) - optional, order in bundle
TableName(string) - Name of the table to create the field on
IndexName(string) - Name of the index to create
Note: the index must exist in the local database
| Example: |
[assembly: DeployCreateField("ACCOUNT", "XAK1ACCOUNT")]
|
DeployCreateTable
| Description: |
Creates a new table in the target database.
| Properties: |
Group(int) - optional, order in bundle
TableName(string) - Name of the table to create the field on
RemoveOnUninstall(boolean) - Indicates whether to remove the table if the bundle is uninstalled
IncludeStructure(boolean) - Indicates whether to create the table structure
IncludeIndicies(boolean) - Indicates whether to create the table indexes
IncludeData(boolean) - Indicates whether to include all data on the table
IncludeJoins(boolean) - Indicates whether to include all joins for the table
Note: the table must exist in the local database
| Example: |
[assembly: DeployCreateTable("MyTable", false, true, true, false, true)]
|
DeployDropField
| Description: |
Drops/removes a field on a target table in the target database.
| Properties: |
Group(int) - optional, order in bundle
TableName(string) - Name of the table where the field exists
FieldName(string) - Name of the field to remove
| Example: |
[assembly: DeployDropField("MyTable", "MyField)]
|
DeployExecuteCommand
| Description: |
Executes a command on the target system when the bundle is installed.
| Properties: |
Group(int) - optional, order in bundle
Command(string) - The command to execute (you can use standard aliases (%App%, %Temp%, %Win%, %Sys%)
WaitForFinish(boolean) - Indicates whether to wait for the command to finish before continuing
| Example: |
[assembly: DeployExecuteCommand("%App%\\System32\\calc.exe", false)]
|
DeployExecuteSQL
| Description: |
Executes a SQL statement on the target database when the bundle is installed.
| Properties: |
Group(int) - optional, order in bundle
SQL(string) - The SQL command to execute
| Example: |
[assembly: DeployExecuteSQL("update account set type = 'Customer' where account like 'Abbott%'")]
|
DeployInsertJoin
| Description: |
Creates a Join in the target database.
| Properties: |
Group(int) - optional, order in bundle
ToTable(string)
ToField(string)
FromTable(string)
FromField(string)
Note: The Join must exist in the local database
| Example: |
[assembly: DeployInsertJoin("Account", "AccountID", "AccountSummary", "AccountID")]
|
DeployInsertLookup
| Description: |
Inserts a Lookup into the target database.
| Properties: |
Group(int) - optional, order in bundle
TableName(string) - The table the Lookup is for
LookupName(string) - The name of the Lookup to include
Note: The Lookup must exist in the local database
| Example: |
[assembly: DeployInsertLookup("Contact", "CONTACT:Lastname")]
|
DeployInsertPickList
| Description: |
Inserts a Picklist into the target database.
| Properties: |
Group(int) - optional, order in bundle
PickListName(string) - The name of the Picklist
IncludeItems(boolean) - optional, Indicates whether to include the items on the Picklist from the local database
ReplaceTargetList(boolean) - optional, Indicates whether the Picklist in the target database should be replaced with the one in the bundle
Note: The Picklist must exist in the local database
| Example: |
[assembly: DeployInsertPickList("Account Type", true, false)]
|
DeployInsertPlugin
| Description: |
Inserts a Plugin into the target database.
| Properties: |
Group(int) - optional, order in bundle
PluginType(PluginType) - The type of plugin (see PluginType enum for plugin types, all types are supported)
Family(string) - The Family where the plugin is in the local database
Name(string) - The name of the plugin in the local database
Company(string) - optional, The company name of the plugin to include
Version(double) - optional, The version of the plugin to include
RemoveOnUninstall(boolean) - optional, Indicates whether to remove the plugin if the bundle is uninstalled
GenerateNewID(boolean) - optional, Indicates whether to generate a new PluginID for the plugin when installed
Note: The Plugin must exist in the local database
| Example: |
[assembly: DeployInsertPlugin(PluginType.ActiveForm, "System", "Account Detail")]
|
DeployInsertRecord
| Description: |
Copies row(s) of data from the local database to the target database.
| Properties: |
Group(int) - optional, order in bundle
TableName(string) - The name of the table to copy the data from in the local database
SourceRecordID(string) - The table ID value of the record to copy
GenerateNewID(string) - optional, Indicates whether to generate new table ID values when copied to the target database
| Example: |
[assembly: DeployInsertRecord("Account", "AXXX00000001", true)]
|
DeployOpenFile
| Description: |
Execute a file on the target system when the bundle is installed.
| Properties: |
Group(int) - optional, order in bundle
TargetFile(string) - The name & path of the file to execute
| Example: |
[assembly: DeployOpenFile("%App%\\Something.bat")]
|
DeployProject
| Description: |
Include the entire contents of a project in Architect in the bundle (how's that for cool?!)
| Properties: |
Group(int) - optional, order in bundle
Project(string) - Name of the project to include
RemoveOnUninstall(boolean) - optional, Indicates whether to remove all included items in the project if the bundle is uninstalled
GenerateNewID(boolean) - optional, Indicates whether to generate new PluginID values for the plugins contained in the project when installed.
| Example: |
[assembly: DeployProject("My Architect Project")]
|
As mentioned before, Attributes can be added to methods, classes, and assemblies. The .NET Extension Deployment attriutes are assembly level attributes only. This really comes down to two things.
- Where you place the attribute. The attribute must not be placed within a class. Usually, you'll find assembly level attributes in the AssemblyInfo.cs file for a C# project (although they do not have to be placed there).
- The attribute must be include the "assembly" prefix.
A small sample class file for a .NET Extension that uses these deployment attributes might look like this:
using System;
using Sage.SalesLogix.NetExtensions;
using Sage.SalesLogix.NetExtensions.Deployment;
[assembly: DeployComment(1, "This is my comment for my bundle")]
[assembly: DeployInsertPickList(2, "Account Type")]
[assembly: DeployCreateTable(2, "MyTable")]
[assembly: DeployProject(2, "My Architect Project")]
[assembly: DeployExecuteSQL(3, "insert into MyTable...")]
namespace MyExtensionSample
{
public class Class1 : Sage.SalesLogix.NetExtensions.BaseRunnable
{
public override object Run(object[] Args)
{
// my extension code here
}
}
}
Now, when I add that .NET Extension file into the .NET Extensions Manager, I can right-click the extension and select view to see the following on the "Deployment" tab:
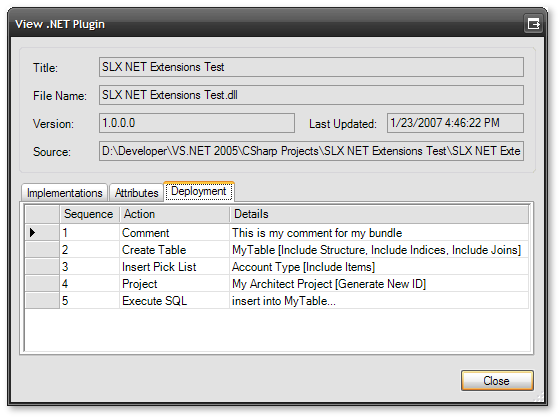
I can now choose to deploy my .NET Extension and create a bundle for it (Choosing "Deploy" in the .NET Extensions Manager for the extension will create a bundle for it), I can open the bundle in Architect and see that the items from my deployment attributes have been included in the bundle!
The deployment attributes feature hasn't received much spotlight (so far) and is often overlooked when talking about the powerful capabilites brought by the .NET Extensions feature in SalesLogix 7, however, once you start to use them, you'll likely wish they existed for other standard plugins built in Architect - I do ;-)
Until next time, happy coding
-Ryan
|
|
|
|
Rate This Article
|
you must log-in to rate articles. [login here] 
|
|
|
Please log in to rate article. |
|
|
Comments & Discussion
|
you must log-in to add comments. [login here]
|
|
|
- subject is missing.
- comment text is missing.
|
|
| Re: A Guide to The .NET Extension Deployment Attributes Posted: 3/1/2007 11:23:12 PM | fiogf49gjkf0d Can't believe I missed this one - nice! | |
|
| Re: A Guide to The .NET Extension Deployment Attributes Posted: 3/2/2007 12:25:21 AM | fiogf49gjkf0d Thanks Dave. You know, this does happen to be the *only* place you'll find this documented as well :-p
-Ryan | |
|
|
|
|
|
Visit the slxdeveloper.com Community Forums!
Not finding the information you need here? Try the forums! Get help from others in the community, share your expertise, get what you need from the slxdeveloper.com community. Go to the forums...
|
|
|
|
|
|